Subqueries and Joins in SQL
Master SQL with our guide on subqueries and joins! Discover how to efficiently combine data from multiple tables, streamline your queries, and enhance performance. Unlock the power of data retrieval through effective SQL techniques.
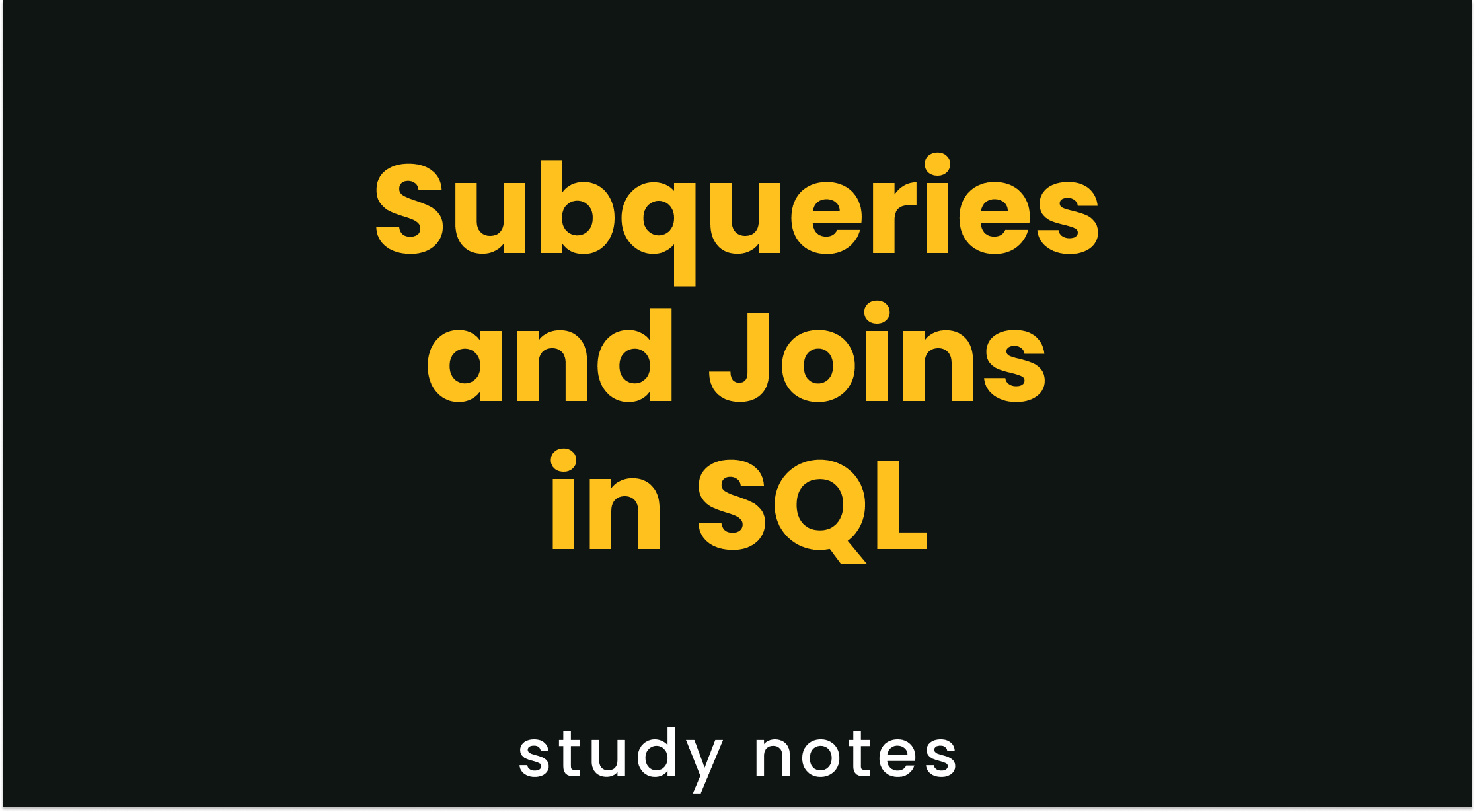
Using Subqueries
- Definition: Subqueries are queries embedded within other queries, allowing for more complex data retrieval from multiple tables
- Purpose: They help combine information from different tables and add filtering criteria from other tables into your main query
- Execution Order: The innermost query is always performed first, then the outer queries build upon those results
- Efficiency: Subqueries can replace multiple separate queries, making the code more concise and easier to maintain
- Use Cases: Particularly useful when:
- Needing to filter based on data from another table
- Combining information from multiple tables
- Creating complex filtering conditions
- Best Practice: When writing subqueries, start with the innermost query and build outward to ensure logical flow
Subquery Best Practices and Considerations
- Unlimited Nesting: Subqueries can be nested multiple times, though this may impact performance
- Single Column Limitation: Subqueries can only retrieve one column at a time in SELECT statements
- Execution Order: Innermost queries execute first, with results feeding into outer queries
- Calculation Capabilities: Subqueries can include calculations and be used for complex aggregations
- Pros:
- Flexible - can be used in SELECT, FROM, WHERE, and HAVING clauses
- Can improve readability by breaking down complex operations
- Can serve as alternatives to joins
- Cons:
- May have performance limitations compared to joins
- Can become complex with multiple nested levels
- Limited functionality (cannot modify data)
- Database support may vary
- Best Practice: Consider performance implications and whether joins might be more appropriate for your specific use case
Joining Tables: An Introduction
- Joins are essential SQL operations that allow combining data from multiple tables in a single query
- Benefits of storing data in multiple tables:
- More efficient storage by avoiding data duplication
- Easier data manipulation and updates
- Better scalability
- Tables are typically organized around business processes or themes
- Key fields serve as critical links between tables, enabling proper record association
- Important characteristics of joins:
- They associate correct records from different tables "on the fly"
- Allow data retrieval from multiple tables in one query
- Are temporary - only exist during query execution
Cartesian (Cross) Joins
- Definition: A Cartesian (Cross) join matches each record from the first table with all records from the second table
- Result Size: The output will have X × Y rows, where X is the number of rows in first table and Y is rows in second table
- Syntax: Use the CROSS JOIN keyword between table names, without need for matching conditions
- Performance Impact: These joins are computationally expensive as they can create very large result sets
- Usage Caution: Should be used carefully as they:
- Can quickly increase data size
- May return incorrect results due to lack of matching criteria
- Are rarely needed in practice
- Example: A table with 29 records joined with a table of 77 records results in 2,233 (29 × 77) records
Inner Joins
- Inner Join Definition: Selects only the records that have matching values in both tables being joined
- Basic Syntax Structure: Use SELECT, FROM, INNER JOIN, and ON clauses to specify the join condition using matching keys
- Column Pre-qualification: Always pre-qualify column names (e.g., table_name.column_name) to avoid ambiguity when the same column name exists in multiple tables
- Multiple Table Joins: You can join multiple tables using successive INNER JOIN statements, but be cautious about performance impact
- Table Aliases: Use meaningful aliases (like 'o' for orders, 'c' for customers) to make code more readable and efficient to write
- Best Practices:
- Avoid unnecessary joins as they impact performance
- Always verify that the returned data matches your expectations
- Use clear and logical table aliases for better code readability
Aliases and Self Joins
- Table Aliases Purpose: Used to simplify and shorten table names in queries, making SQL code more readable and easier to write
- Alias Duration: Only exists for the duration of the query execution - does not permanently rename tables
- Naming Conventions: Can use single letters (v, p), abbreviated names (ven, prod), or any logical shorthand that makes sense
- Self Joins: Allow joining a table to itself, useful for hierarchical data like employee-manager relationships
- Alias Necessity: Required when performing self joins to distinguish between different instances of the same table
- Practical Applications: Particularly valuable in organizational structures, reporting hierarchies, and relationship mapping within the same dataset
Advanced Joins: Left, Right, and Full Outer Joins
- Left Join: Returns all records from the left table (first table mentioned) and matching records from the right table. If no match exists, NULL values are returned for the right table.
- Right Join: Returns all records from the right table (second table mentioned) and matching records from the left table. Not supported in SQLite, but can be achieved by reversing table order in a LEFT JOIN.
- Full Outer Join: Returns all records when there's a match in either left or right table. Not supported in SQLite.
- Table Order Matters: The order of tables in the FROM and JOIN clauses affects the results when using LEFT or RIGHT joins.
- SQLite Limitations: Only supports LEFT JOIN among these three types, but RIGHT JOIN functionality can be achieved by reordering tables.
- Use Case Example: LEFT JOIN is useful when you want to see all customers whether they have placed orders or not.
Unions
- Purpose: UNION combines results from two or more queries into a single table
- Requirements for UNION:
- Same number of columns in each query
- Similar data types across corresponding columns
- Columns must be in the same order
- Syntax: Write first SELECT statement, then UNION keyword, followed by second SELECT statement
- Use Cases: Particularly useful when you need to combine similar data from different tables into one comprehensive list
- Best Practice: While not used daily, it's a valuable tool for specific scenarios where data needs to be vertically combined
Example syntax:
SELECT column1, column2 FROM table1
UNION
SELECT column1, column2 FROM table2;
Summary
- Start Simple and Verify: Begin with one table, verify results, then add more tables gradually. Check record counts after each join.
- Plan Before Coding: Take time to visualize and plan how tables will merge before writing queries. Drawing table relationships can help.
- Join Conditions are Essential: Always use join conditions for inner, left, and right joins (except for Cartesian joins and unions).
- Performance Awareness: More table joins = worse performance. Only retrieve the data you actually need for analysis.
- Check for Data Issues: Monitor for unexpected duplicates and verify record counts match expectations.
- Inner Joins are Most Common: While inner joins are most frequently used, understanding all join types is valuable.
- Database Compatibility: Be aware that join syntax may vary between different database management systems.
Visual join diagrams can be helpful references when learning and implementing different types of SQL joins.